Test Time Compute
Published 12/13/2024
Written by Ken Huang, CEO of DistributedApps.ai and VP of Research at CSA GCR.
Everyone seems to talk about Test-Time Computation or Test Time Compute (TTC) as a way to scale the reasoning capability of large language models (LLMs). What is it about and why is it important now? This blog post is an attempt to answer this question.
Key Aspects of Test-Time Computation
Inference Process
During TTC, the model takes input data and applies its learned parameters to produce an output. For neural networks, this involves forward propagation through the network layers, using matrix multiplications and activation functions.
Computational Resources
TTC directly impacts the responsiveness and efficiency of AI systems in real-world applications. The computational intensity can affect scalability and user satisfaction, especially in time-sensitive environments like autonomous vehicles or real-time analytics systems.
Advanced Test-Time Compute Strategies
Recent advancements have explored various methods to enhance model performance during inference without retraining:
Adaptive Distribution Updates
The model's distribution over responses is updated adaptively at test time, allowing for iterative refinement of outputs.
Compute-Optimal Scaling
This strategy allocates test-time compute resources adaptively based on the difficulty of the prompt, significantly improving efficiency compared to traditional methods.
Reward Modeling
In test-time compute, reward modeling helps rank and evaluate multiple outputs generated by the model during inference. Instead of being limited to a single output, the model generates several candidates, and the reward model scores these outputs based on predefined criteria. This allows the system to select the output that best aligns with the desired objective, improving quality and relevance.
The Figure below illustrates a workflow for generating, evaluating, and selecting outputs in an AI system. The process appears to follow a reward-based ranking system, commonly seen in techniques such as reinforcement learning and test-time compute optimization. Here's a detailed explanation of the workflow:
- Model Generation: At the beginning, a model is tasked with generating multiple outputs based on a given input. These outputs represent different possible responses or solutions produced by the model.
- Multiple Outputs (N Outputs): The model generates nn outputs, labeled as Output 1, Output 2, Output 3, ..., Output n. Each output represents one candidate solution or response for the given task. This step ensures diversity in the generated results, providing a range of options that can later be evaluated.
- Reward Model Evaluation: A reward model is used to evaluate each of the nn outputs. The reward model assigns a score to each output based on how well it aligns with a predefined objective or criterion. The evaluation considers aspects like relevance, correctness, fluency, or adherence to specific requirements. The reward model essentially quantifies the desirability of each output.
- Ranking Mechanism: Based on the scores provided by the reward model, the outputs are ranked. The outputs are ordered such that the one with the highest score is ranked first, followed by the second-highest, and so on. This ranking process ensures that the most optimal output, according to the reward model, is identified.
- Final Selection: The top-ranked output (in this case, Output 3, as indicated by the diagram) is selected as the final result. This output is then provided as the system's response or solution to the given task.
Figure 1: Reward Model during TTC Reasoning
Self Verification
Self-verification enables AI systems to validate the quality and correctness of their outputs during inference. In this context, the model may generate an output and then evaluate it using internal checks, such as ensuring logical consistency or adherence to task-specific constraints. This iterative approach helps refine the output without requiring external validation.
Search Methods
Test-time compute often involves using search methods to explore possible solutions dynamically. Techniques like beam search or tree-based exploration (e.g., Monte Carlo Tree Search) allow the system to evaluate multiple paths or outputs, enabling it to find the most optimal solution within computational constraints. This approach is commonly used in generative tasks like language modeling or game decision-making.
Best-of-N Sampling
This method is a cornerstone of test-time compute, where the model generates NN outputs for a single input, and the system evaluates them to select the best candidate. This approach trades additional computational cost at inference for improved output quality, ensuring that the selected result is the most appropriate or high-performing option based on a scoring function or ranking mechanism.
STaR Algorithm (Self-Taught Reasoner)
In test-time compute, the STaR algorithm can iteratively refine a model's reasoning during inference by generating multiple solutions, evaluating them, and incorporating feedback. This feedback loop at test time enables the model to adjust its reasoning and improve output accuracy on the fly, especially for complex or ambiguous tasks.
Verifier
A verifier is employed at test time to assess the reliability and correctness of a model's output. This secondary system evaluates whether the generated result meets certain criteria, such as being logically valid, free from contradictions, or adhering to task-specific requirements. For instance, in code generation, the verifier might simulate code execution to ensure correctness before outputting the result.
Monte Carlo Tree Search (MCTS)
In test-time compute, MCTS is used to explore and evaluate multiple potential decisions or outputs by building a search tree dynamically during inference. It balances exploration (testing less familiar options) and exploitation (focusing on the most promising options) to identify near-optimal solutions efficiently. This technique is particularly effective in tasks requiring strategic decision-making, such as games or planning problems.
These methods collectively enable AI systems to leverage additional computational resources during inference to achieve higher-quality outputs. By incorporating techniques like sampling, ranking, and verification at test time, systems can dynamically refine their results and perform better on complex or nuanced tasks without modifying the underlying model architecture. This paradigm enhances the flexibility and effectiveness of AI models across a wide range of applications.
Implications and Future Directions
Optimizing test-time compute has the potential to be more effective than scaling up model size through additional pretraining. This finding has important implications for the future of LLM development and deployment strategies.
Adaptive Strategies
The effectiveness of test-time compute strategies varies depending on problem difficulty. Easier problems may benefit from iterative refinement, while harder problems might require broader exploration of solution spaces.
Balancing Pre-Training and Inference
Future AI systems may shift towards allocating more resources to test-time computation rather than focusing solely on scaling up pre-training. This could lead to more efficient and adaptable models.
Code Example
The following example integrates the LLaMA-3 model with Monte Carlo Tree Search (MCTS) for TTC reasoning, you could follow these steps:
- Model Setup: Load the pre-trained LLaMA-3 model.
- State Evaluation: Use the LLaMA-3 model to evaluate game states or decision points during MCTS.
- MCTS Integration: Use MCTS to explore possible moves, with the LLaMA-3 model providing evaluations of states.
- Decision Making: Select moves based on MCTS results combined with LLaMA-3 evaluations.
Here's a conceptual example in Python:
1: Import necessary libraries
import torch from transformers import AutoTokenizer, AutoModelForCausalLM
2: Load LLaMA-3 model
tokenizer = AutoTokenizer.from_pretrained("meta-llama/Llama-3-8B") model = AutoModelForCausalLM.from_pretrained("meta-llama/Llama-3-8B")
3: Function to evaluate game states
def evaluate_state(state): input_ids = tokenizer.encode(state, return_tensors="pt") with torch.no_grad(): output = model(input_ids) return output.logits.mean().item()
4: Define MCTS Node Class
class MCTSNode: def __init__(self, state, parent=None): self.state = state self.parent = parent self.children = [] self.visits = 0 self.value = 0 def expand(self): # Placeholder for expanding nodes (generate possible moves) pass def simulate(self): # Use LLaMA-3 model to evaluate the state return evaluate_state(self.state) def backpropagate(self, result): self.visits += 1 self.value += result if self.parent: self.parent.backpropagate(result)
5: Example ofMCTS integration
root = MCTSNode("initial_state") for _ in range(100): # Selection and Expansion current_node = root while current_node.children: current_node = np.random.choice(current_node.children) current_node.expand() # Simulation result = current_node.simulate() # Backpropagation current_node.backpropagate(result) # Select best move based on MCTS results best_move = max(root.children, key=lambda c: c.value / c.visits)
This example shows a simplified integration of the LLaMA-3 model with MCTS for state evaluation during decision-making.The LLaMA-3 model evaluates states, while MCTS explores and selects the best moves.
Why TTC is important
Here are reasons why it has become important now.
1. Performance Improvement: TTC can significantly enhance model performance without requiring additional training. On the challenging MATH dataset, researchers observed up to 21.6% improvement in accuracy on the test set compared to traditional methods(Snell et al., 2024).
2. Efficiency Gains: Optimizing TTC can lead to substantial efficiency improvements. Snell et al. (2024) found that their "compute-optimal" strategy improved efficiency by more than 4x compared to traditional best-of-N sampling approaches.
3. Scalability Alternative: TTC offers an alternative to simply scaling up model parameters. In some cases, smaller models enhanced with optimized TTC outperformed models 14x larger that didn't utilize additional computation at test time.
4. Adaptive Problem-Solving: TTC enables models to allocate computational resources based on problem difficulty, mimicking human behavior of spending more time on challenging tasks.
5. Future AI Development: The research on TTC challenges the "bigger is better" paradigm in AI development, potentially leading to more efficient and cost-effective AI systems.
6. Self-Improvement Capability: Enabling LLMs to improve their outputs using more test-time computation is a critical step towards building generally self-improving agents that can operate on open-ended natural language tasks).
These findings highlight the importance of TTC in advancing the capabilities and efficiency of large language models, potentially reshaping future approaches to AI development and deployment.
References
Snell, C., Kostrikov, I., Sutskever, I., & Szlam, A. (2024). Scaling LLM Test-Time Compute Optimally can be More Effective than Scaling Model Parameters. arXiv preprint arXiv:2408.03314.
Related Resources
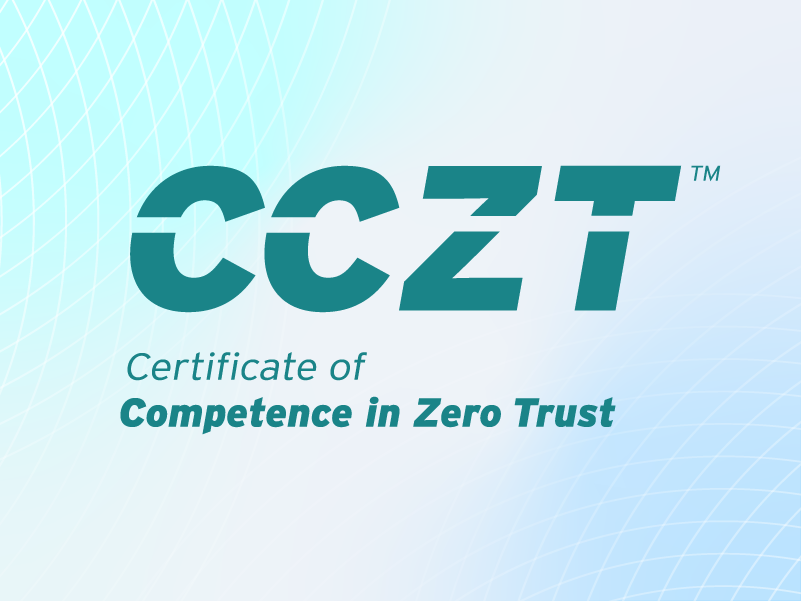
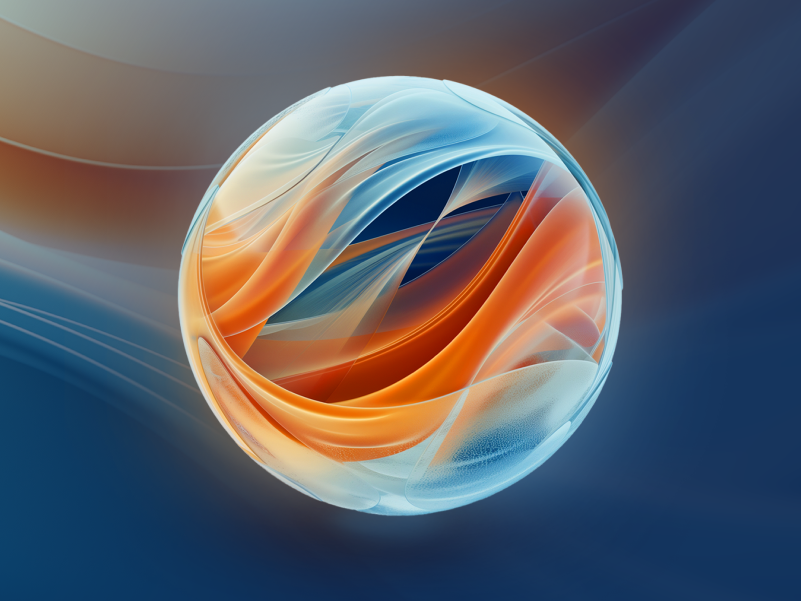
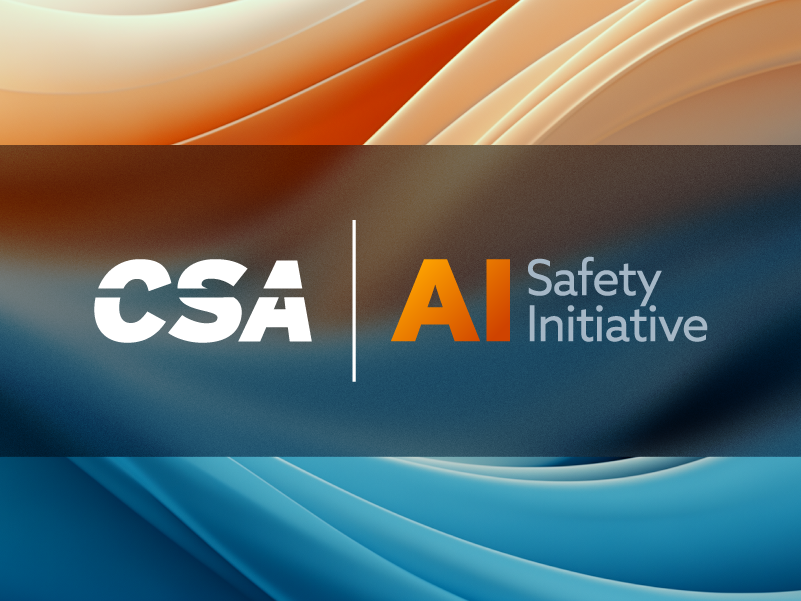
Related Articles:
Next-Gen Cybersecurity with AI: Reshaping Digital Defense
Published: 01/10/2025
The Rise of Malicious AI: 5 Key Insights from an Ethical Hacker
Published: 01/03/2025
The EU AI Act and SMB Compliance
Published: 12/18/2024
Zero-Code Cloud: Building Secure, Automated Infrastructure Without Writing a Line
Published: 12/16/2024